不错呦!smile@林凯西,确保“准备文件”中的几个文件都有安装,S...您好,看了您这篇帖子觉得很有帮助。但是有个问题想请...我的修改过了怎么还被恶意注册呢 @jjjjiiii 用PJ快9年了,主要是A...PJ3啊,貌似很少有人用PJ了,现在不是WP就是z...@332347365,我当时接入时错误码没有-10...楼主,ChkValue值应为-103是什么意思呢?...大哥 你最近能看到我发的信息,请跟我联系,我有个制...
Entity Framework添加导航属性运行时提示生成Model失败解决方法
编辑:dnawo 日期:2012-09-11
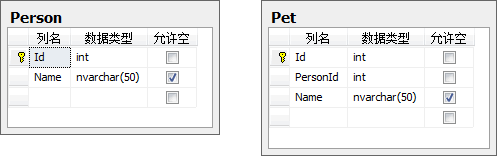
项目使用Entity Framework Power Tools Beta 2生成Models,后来在Pet.PersonId和Petson.Id间添加了主外键, 因为有手工修改过Models,不能用工具重新生成,只能手工添加导航属性相关代码:
Person.cs:
复制内容到剪贴板
程序代码

using System;
using System.Collections.Generic;
namespace ConsoleApplication1.Models
{
public class Person
{
public Person()
{
this.Pets = new List<Pet>();
}
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<Pet> Pets { get; set; }
}
}
using System.Collections.Generic;
namespace ConsoleApplication1.Models
{
public class Person
{
public Person()
{
this.Pets = new List<Pet>();
}
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<Pet> Pets { get; set; }
}
}
Pet.cs:
复制内容到剪贴板
程序代码

using System;
using System.Collections.Generic;
namespace ConsoleApplication1.Models
{
public class Pet
{
public int Id { get; set; }
public int PersonId { get; set; }
public string Name { get; set; }
public virtual Person Person { get; set; }
}
}
using System.Collections.Generic;
namespace ConsoleApplication1.Models
{
public class Pet
{
public int Id { get; set; }
public int PersonId { get; set; }
public string Name { get; set; }
public virtual Person Person { get; set; }
}
}
PetMap.cs:
复制内容到剪贴板
程序代码

using System.ComponentModel.DataAnnotations;
using System.Data.Entity.ModelConfiguration;
namespace ConsoleApplication1.Models.Mapping
{
public class PetMap : EntityTypeConfiguration<Pet>
{
public PetMap()
{
// Primary Key
this.HasKey(t => t.Id);
// Properties
this.Property(t => t.Name)
.HasMaxLength(50);
// Table & Column Mappings
this.ToTable("Pet");
this.Property(t => t.Id).HasColumnName("Id");
this.Property(t => t.PersonId).HasColumnName("PersonId");
this.Property(t => t.Name).HasColumnName("Name");
this.HasOptional(t => t.Person)
.WithMany(t => t.Pets)
.HasForeignKey(d => d.PersonId);
}
}
}
using System.Data.Entity.ModelConfiguration;
namespace ConsoleApplication1.Models.Mapping
{
public class PetMap : EntityTypeConfiguration<Pet>
{
public PetMap()
{
// Primary Key
this.HasKey(t => t.Id);
// Properties
this.Property(t => t.Name)
.HasMaxLength(50);
// Table & Column Mappings
this.ToTable("Pet");
this.Property(t => t.Id).HasColumnName("Id");
this.Property(t => t.PersonId).HasColumnName("PersonId");
this.Property(t => t.Name).HasColumnName("Name");
this.HasOptional(t => t.Person)
.WithMany(t => t.Pets)
.HasForeignKey(d => d.PersonId);
}
}
}
运行报错,提示:

One or more validation errors were detected during model generation:
System.Data.Edm.EdmAssociationType: : Multiplicity conflicts with the referential constraint in Role 'Pet_Person_Target' in relationship 'Pet_Person'. Because all of the properties in the Dependent Role are non-nullable, multiplicity of the Principal Role must be '1'.
System.Data.Edm.EdmAssociationType: : Multiplicity conflicts with the referential constraint in Role 'Pet_Person_Target' in relationship 'Pet_Person'. Because all of the properties in the Dependent Role are non-nullable, multiplicity of the Principal Role must be '1'.
经多次测试比较,最终发现PetMap类需做如下修改:
复制内容到剪贴板
程序代码

this.HasRequired(t => t.Person)
.WithMany(t => t.Pets)
.HasForeignKey(d => d.PersonId);
.WithMany(t => t.Pets)
.HasForeignKey(d => d.PersonId);
HasOptional和HasRequired的区别:

HasOptional:Configures an optional relationship from this entity type. Instances of the entity type will be able to be saved to the database without this relationship being specified. The foreign key in the database will be nullable.
HasRequired:Configures a required relationship from this entity type. Instances of the entity type will not be able to be saved to the database unless this relationship is specified. The foreign key in the database will be non-nullable.
HasRequired:Configures a required relationship from this entity type. Instances of the entity type will not be able to be saved to the database unless this relationship is specified. The foreign key in the database will be non-nullable.
后记:最开始以为这个错误和表字段允许空有关,其实是没有关系的,它更多和Pet类PersonId属性的类型有关系,若PersonId类型为Nullable<int>,则不会有上述错误。






评论: 0 | 引用: 0 | 查看次数: 6126
发表评论
请登录后再发表评论!