不错呦!smile@林凯西,确保“准备文件”中的几个文件都有安装,S...您好,看了您这篇帖子觉得很有帮助。但是有个问题想请...我的修改过了怎么还被恶意注册呢 @jjjjiiii 用PJ快9年了,主要是A...PJ3啊,貌似很少有人用PJ了,现在不是WP就是z...@332347365,我当时接入时错误码没有-10...楼主,ChkValue值应为-103是什么意思呢?...大哥 你最近能看到我发的信息,请跟我联系,我有个制...
一种窗体间传值简单方法
编辑:dnawo 日期:2009-10-20
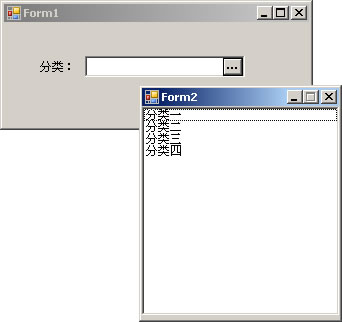
上图所示的窗体传值示例,在windows应用程序中经常都会碰到,以前是想办法将form1对象传给form2,然后在form2中在适当的时机调用form1对象上的控件并设置值:
Form1.cs:
复制内容到剪贴板
程序代码

using System;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
/// <summary>
/// 新增属性,设置textBox1的文本,供Form2调用
/// </summary>
public string Value
{
set { textBox1.Text = value; }
}
private void button1_Click(object sender, EventArgs e)
{
(new Form2()).Show(this);//Form1传给Form2
}
}
}
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
/// <summary>
/// 新增属性,设置textBox1的文本,供Form2调用
/// </summary>
public string Value
{
set { textBox1.Text = value; }
}
private void button1_Click(object sender, EventArgs e)
{
(new Form2()).Show(this);//Form1传给Form2
}
}
}
Form2.cs:
复制内容到剪贴板
程序代码

using System;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
/// <summary>
/// 双击选择
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void listBox1_DoubleClick(object sender, EventArgs e)
{
Form frm = this.Owner;
if (frm is Form1)
((Form1)frm).Value = listBox1.SelectedItem.ToString();
this.Close();
}
}
}
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
/// <summary>
/// 双击选择
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void listBox1_DoubleClick(object sender, EventArgs e)
{
Form frm = this.Owner;
if (frm is Form1)
((Form1)frm).Value = listBox1.SelectedItem.ToString();
this.Close();
}
}
}
今天在一个项目中发现了另一种方法,更为简便:
Form1.cs:
复制内容到剪贴板
程序代码

using System;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
Form2 frm = new Form2();
textBox1.Text = frm.GetValue();
}
}
}
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
Form2 frm = new Form2();
textBox1.Text = frm.GetValue();
}
}
}
Form2.cs:
复制内容到剪贴板
程序代码

using System;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
/// <summary>
/// 获取文本框中的值
/// </summary>
/// <returns></returns>
public string GetValue()
{
this.ShowDialog();//一定不能用this.Show()
//下边代码得this.Close()后才能继续执行
if (listBox1.SelectedIndex != -1)
return listBox1.SelectedItem.ToString();
else
return string.Empty;
}
/// <summary>
/// 双击选择
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void listBox1_DoubleClick(object sender, EventArgs e)
{
this.Close();
}
}
}
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
/// <summary>
/// 获取文本框中的值
/// </summary>
/// <returns></returns>
public string GetValue()
{
this.ShowDialog();//一定不能用this.Show()
//下边代码得this.Close()后才能继续执行
if (listBox1.SelectedIndex != -1)
return listBox1.SelectedItem.ToString();
else
return string.Empty;
}
/// <summary>
/// 双击选择
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void listBox1_DoubleClick(object sender, EventArgs e)
{
this.Close();
}
}
}
评论: 0 | 引用: 0 | 查看次数: 3386
发表评论
请登录后再发表评论!