不错呦!smile@林凯西,确保“准备文件”中的几个文件都有安装,S...您好,看了您这篇帖子觉得很有帮助。但是有个问题想请...我的修改过了怎么还被恶意注册呢 @jjjjiiii 用PJ快9年了,主要是A...PJ3啊,貌似很少有人用PJ了,现在不是WP就是z...@332347365,我当时接入时错误码没有-10...楼主,ChkValue值应为-103是什么意思呢?...大哥 你最近能看到我发的信息,请跟我联系,我有个制...
Google Maps JavaScript API V3拉框画矩形实现代码
编辑:dnawo 日期:2011-05-19
Google Maps JavaScript API V3中没有提供相关的类或方法来实现拉框画矩形,最终的实现代码是基于演示库中的两个例子完成的。第一个例子是Rectangle Overlay,它让我知道有Rectangle这个类,翻遍Google Maps JavaScript API V3 文档,只有在API参考中有提到它,这让我知道看完整个文档是多么重要;第二个例子是KeyDragZoom,它实现了在地图上拉框并移动地图以这个框为中心,我的代码主要是在这个例子上完成的,去掉了原有的移动地图相关部分,并在拉框后在地图上添加了一个Rectangle,最终效果如下图所示:
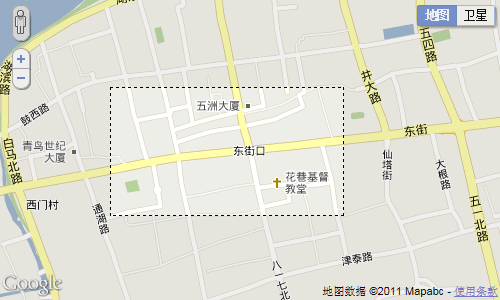
首先需修改下keydragzoom.js,使拉框后地图不移动(将红色部分注释即可):
剩下的就很简单了,直接给出完整代码:
好了,打开页面待地图加载完成后,按住shift键,就可以拉框画矩形了!
相关资源
·Rectangle Overlay:http://gmaps-samples-v3.googlecode.com/svn/trunk/rectangle-overlay/rectangle-overlay.html
·KeyDragZoom:http://google-maps-utility-library-v3.googlecode.com/svn/tags/keydragzoom/2.0.5/docs/examples.html
·keydragzoom.js:http://www.mzwu.com/pic/201105/keydragzoom.rar
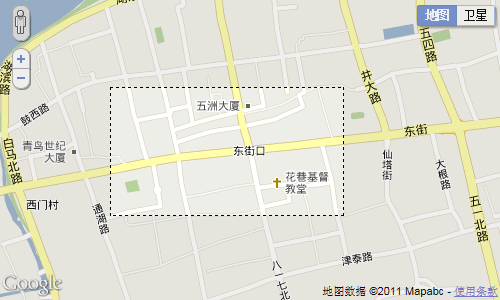
首先需修改下keydragzoom.js,使拉框后地图不移动(将红色部分注释即可):
复制内容到剪贴板
程序代码

DragZoom.prototype.onMouseUp_ = function (e) {
var z;
var me = this;
this.mouseDown_ = false;
if (this.dragging_) {
if ((this.getMousePoint_(e).x === this.startPt_.x) && (this.getMousePoint_(e).y === this.startPt_.y)) {
this.onKeyUp_(e); // Cancel event
return;
}
var left = Math.min(this.startPt_.x, this.endPt_.x);
var top = Math.min(this.startPt_.y, this.endPt_.y);
var width = Math.abs(this.startPt_.x - this.endPt_.x);
var height = Math.abs(this.startPt_.y - this.endPt_.y);
// Google Maps API bug: setCenter() doesn't work as expected if the map has a
// border on the left or top. The code here includes a workaround for this problem.
var kGoogleCenteringBug = true;
if (kGoogleCenteringBug) {
left += this.borderWidths_.left;
top += this.borderWidths_.top;
}
var prj = this.prjov_.getProjection();
var sw = prj.fromContainerPixelToLatLng(new google.maps.Point(left, top + height));
var ne = prj.fromContainerPixelToLatLng(new google.maps.Point(left + width, top));
var bnds = new google.maps.LatLngBounds(sw, ne);
// Sometimes fitBounds causes a zoom OUT, so restore original zoom level if this happens.
z = this.map_.getZoom();
//this.map_.fitBounds(bnds);
if (this.map_.getZoom() < z) {
this.map_.setZoom(z);
}
// Redraw box after zoom:
var swPt = prj.fromLatLngToContainerPixel(sw);
var nePt = prj.fromLatLngToContainerPixel(ne);
if (kGoogleCenteringBug) {
swPt.x -= this.borderWidths_.left;
swPt.y -= this.borderWidths_.top;
nePt.x -= this.borderWidths_.left;
nePt.y -= this.borderWidths_.top;
}
this.boxDiv_.style.left = swPt.x + "px";
this.boxDiv_.style.top = nePt.y + "px";
this.boxDiv_.style.width = (Math.abs(nePt.x - swPt.x) - (this.boxBorderWidths_.left + this.boxBorderWidths_.right)) + "px";
this.boxDiv_.style.height = (Math.abs(nePt.y - swPt.y) - (this.boxBorderWidths_.top + this.boxBorderWidths_.bottom)) + "px";
// Hide box asynchronously after 1 second:
setTimeout(function () {
me.boxDiv_.style.display = "none";
}, 1000);
this.dragging_ = false;
this.onMouseMove_(e); // Updates the veil
/**
* This event is fired when the drag operation ends.
* The parameter passed is the geographic bounds of the selected area.
* Note that this event is <i>not</i> fired if the hot key is released before the drag operation ends.
* @name DragZoom#dragend
* @param {LatLngBounds} bnds The geographic bounds of the selected area.
* @event
*/
google.maps.event.trigger(this, "dragend", bnds);
// if the hot key isn't down, the drag zoom must have been activated by turning
// on the visual control. In this case, finish up by simulating a key up event.
if (!this.isHotKeyDown_(e)) {
this.onKeyUp_(e);
}
}
};
var z;
var me = this;
this.mouseDown_ = false;
if (this.dragging_) {
if ((this.getMousePoint_(e).x === this.startPt_.x) && (this.getMousePoint_(e).y === this.startPt_.y)) {
this.onKeyUp_(e); // Cancel event
return;
}
var left = Math.min(this.startPt_.x, this.endPt_.x);
var top = Math.min(this.startPt_.y, this.endPt_.y);
var width = Math.abs(this.startPt_.x - this.endPt_.x);
var height = Math.abs(this.startPt_.y - this.endPt_.y);
// Google Maps API bug: setCenter() doesn't work as expected if the map has a
// border on the left or top. The code here includes a workaround for this problem.
var kGoogleCenteringBug = true;
if (kGoogleCenteringBug) {
left += this.borderWidths_.left;
top += this.borderWidths_.top;
}
var prj = this.prjov_.getProjection();
var sw = prj.fromContainerPixelToLatLng(new google.maps.Point(left, top + height));
var ne = prj.fromContainerPixelToLatLng(new google.maps.Point(left + width, top));
var bnds = new google.maps.LatLngBounds(sw, ne);
// Sometimes fitBounds causes a zoom OUT, so restore original zoom level if this happens.
z = this.map_.getZoom();
//this.map_.fitBounds(bnds);
if (this.map_.getZoom() < z) {
this.map_.setZoom(z);
}
// Redraw box after zoom:
var swPt = prj.fromLatLngToContainerPixel(sw);
var nePt = prj.fromLatLngToContainerPixel(ne);
if (kGoogleCenteringBug) {
swPt.x -= this.borderWidths_.left;
swPt.y -= this.borderWidths_.top;
nePt.x -= this.borderWidths_.left;
nePt.y -= this.borderWidths_.top;
}
this.boxDiv_.style.left = swPt.x + "px";
this.boxDiv_.style.top = nePt.y + "px";
this.boxDiv_.style.width = (Math.abs(nePt.x - swPt.x) - (this.boxBorderWidths_.left + this.boxBorderWidths_.right)) + "px";
this.boxDiv_.style.height = (Math.abs(nePt.y - swPt.y) - (this.boxBorderWidths_.top + this.boxBorderWidths_.bottom)) + "px";
// Hide box asynchronously after 1 second:
setTimeout(function () {
me.boxDiv_.style.display = "none";
}, 1000);
this.dragging_ = false;
this.onMouseMove_(e); // Updates the veil
/**
* This event is fired when the drag operation ends.
* The parameter passed is the geographic bounds of the selected area.
* Note that this event is <i>not</i> fired if the hot key is released before the drag operation ends.
* @name DragZoom#dragend
* @param {LatLngBounds} bnds The geographic bounds of the selected area.
* @event
*/
google.maps.event.trigger(this, "dragend", bnds);
// if the hot key isn't down, the drag zoom must have been activated by turning
// on the visual control. In this case, finish up by simulating a key up event.
if (!this.isHotKeyDown_(e)) {
this.onKeyUp_(e);
}
}
};
剩下的就很简单了,直接给出完整代码:
复制内容到剪贴板
程序代码

<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Rectangle</title>
</head>
<body onload="initialize()">
<div id="map_canvas" style="width:500px; height:300px;"></div>
<script type="text/javascript" src="http://maps.google.com/maps/api/js?sensor=false"></script>
<script src="Scripts/keydragzoom.js" type="text/javascript"></script>
<script type="text/javascript">
function initialize(){
var myOptions = {
center : new google.maps.LatLng(26.085963630752868, 119.29929775619507),
zoom : 15,
mapTypeId : google.maps.MapTypeId.ROADMAP
};
var map = new google.maps.Map(document.getElementById("map_canvas"), myOptions);
var rectangle = new google.maps.Rectangle({
map : map,
bounds : new google.maps.LatLngBounds(map.getCenter(),map.getCenter()),
strokeColor : "black",
strokeWeight : "1",
strokeOpacity : 1,
fillColor : "#E6ECEA",
fillOpacity : 0.5
});
map.enableKeyDragZoom({
key: "shift",
boxStyle: {
border: "1px dashed black",
backgroundColor: "transparent",
opacity: 1
},
veilStyle: {
backgroundColor: "gray",
opacity: 0.1
},
visualEnabled: true,
visualPosition: google.maps.ControlPosition.LEFT,
visualPositionOffset: new google.maps.Size(12, 0),
visualPositionIndex: null,
visualSprite: "http://www.mzwu.com/pic/201105/dragzoom_btn.png",
visualSize: new google.maps.Size(20, 20),
visualTips: {
off: "Turn on",
on: "Turn off"
}
});
var dz = map.getDragZoomObject();
google.maps.event.addListener(dz, "dragend", function(bnds){
rectangle.setBounds(bnds);
});
}
</script>
</body>
</html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Rectangle</title>
</head>
<body onload="initialize()">
<div id="map_canvas" style="width:500px; height:300px;"></div>
<script type="text/javascript" src="http://maps.google.com/maps/api/js?sensor=false"></script>
<script src="Scripts/keydragzoom.js" type="text/javascript"></script>
<script type="text/javascript">
function initialize(){
var myOptions = {
center : new google.maps.LatLng(26.085963630752868, 119.29929775619507),
zoom : 15,
mapTypeId : google.maps.MapTypeId.ROADMAP
};
var map = new google.maps.Map(document.getElementById("map_canvas"), myOptions);
var rectangle = new google.maps.Rectangle({
map : map,
bounds : new google.maps.LatLngBounds(map.getCenter(),map.getCenter()),
strokeColor : "black",
strokeWeight : "1",
strokeOpacity : 1,
fillColor : "#E6ECEA",
fillOpacity : 0.5
});
map.enableKeyDragZoom({
key: "shift",
boxStyle: {
border: "1px dashed black",
backgroundColor: "transparent",
opacity: 1
},
veilStyle: {
backgroundColor: "gray",
opacity: 0.1
},
visualEnabled: true,
visualPosition: google.maps.ControlPosition.LEFT,
visualPositionOffset: new google.maps.Size(12, 0),
visualPositionIndex: null,
visualSprite: "http://www.mzwu.com/pic/201105/dragzoom_btn.png",
visualSize: new google.maps.Size(20, 20),
visualTips: {
off: "Turn on",
on: "Turn off"
}
});
var dz = map.getDragZoomObject();
google.maps.event.addListener(dz, "dragend", function(bnds){
rectangle.setBounds(bnds);
});
}
</script>
</body>
</html>
好了,打开页面待地图加载完成后,按住shift键,就可以拉框画矩形了!
相关资源
·Rectangle Overlay:http://gmaps-samples-v3.googlecode.com/svn/trunk/rectangle-overlay/rectangle-overlay.html
·KeyDragZoom:http://google-maps-utility-library-v3.googlecode.com/svn/tags/keydragzoom/2.0.5/docs/examples.html
·keydragzoom.js:http://www.mzwu.com/pic/201105/keydragzoom.rar
评论: 0 | 引用: 0 | 查看次数: 12307
发表评论
请登录后再发表评论!