不错呦!smile@林凯西,确保“准备文件”中的几个文件都有安装,S...您好,看了您这篇帖子觉得很有帮助。但是有个问题想请...我的修改过了怎么还被恶意注册呢 @jjjjiiii 用PJ快9年了,主要是A...PJ3啊,貌似很少有人用PJ了,现在不是WP就是z...@332347365,我当时接入时错误码没有-10...楼主,ChkValue值应为-103是什么意思呢?...大哥 你最近能看到我发的信息,请跟我联系,我有个制...
Entity Framework 4.3简单使用示例
编辑:dnawo 日期:2012-08-08
第一步:在项目中引用程序集
·EntityFramework
·System.ComponentModel.DataAnnotations
·System.Data.Entity
第二步:在App.config文件配置数据库连接字符串
引用内容
第三步:编写程序
①.编写实体类:
②.编写一个DbContext子类:
③.使用示例:
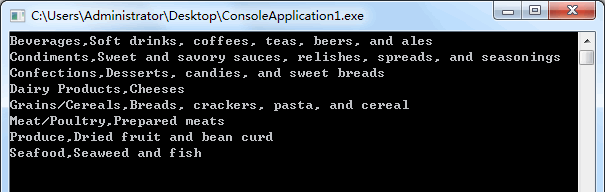
补充说明
[1].实体类主键必须有[Key]约束,否则程序运行会出错:
引用内容
[2].如果实体类名和表名不一致,必须指定表名:
[3].实体类属性只能少于或等于表字段,否则添加记录会出错;
·EntityFramework
·System.ComponentModel.DataAnnotations
·System.Data.Entity
第二步:在App.config文件配置数据库连接字符串

<add name="SQLServer" providerName="System.Data.SqlClient" connectionString="Data Source=localhost;Initial Catalog=Northwind;Pooling=False;user=sa;pwd=sa;" />
第三步:编写程序
①.编写实体类:
复制内容到剪贴板
程序代码

using System;
using System.ComponentModel.DataAnnotations;
namespace ConsoleApplication1
{
public class Categories
{
[Key]
public int CategoryID { get; set; }
public string CategoryName { get; set; }
public string Description { get; set; }
public byte[] Picture { get; set; }
}
}
using System.ComponentModel.DataAnnotations;
namespace ConsoleApplication1
{
public class Categories
{
[Key]
public int CategoryID { get; set; }
public string CategoryName { get; set; }
public string Description { get; set; }
public byte[] Picture { get; set; }
}
}
②.编写一个DbContext子类:
复制内容到剪贴板
程序代码

using System;
using System.Data.Entity;
namespace ConsoleApplication1
{
public class CAContext : DbContext
{
public CAContext()
: base("Name=SQLServer")
{ }
public DbSet<Categories> Categories { get; set; }
//继续添加多个实体类...
}
}
using System.Data.Entity;
namespace ConsoleApplication1
{
public class CAContext : DbContext
{
public CAContext()
: base("Name=SQLServer")
{ }
public DbSet<Categories> Categories { get; set; }
//继续添加多个实体类...
}
}
③.使用示例:
复制内容到剪贴板
程序代码

using System;
using System.Linq;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
CAContext context = new CAContext();
Categories entity;
//添加
entity = new Categories() { CategoryID = 0, CategoryName = "fruit", Description = "-", Picture = null };
context.Categories.Add(entity);
context.SaveChanges();
//修改
entity = context.Categories.Where(c => c.CategoryName == "fruit").First();
if (entity != null)
{
entity.Description = "fruit description.";
context.SaveChanges();
}
//删除
entity = context.Categories.Where(c => c.CategoryName == "fruit").First();
if (entity != null)
{
context.Categories.Remove(entity);
context.SaveChanges();
}
//查询
var categories = context.Categories;
foreach (var item in categories)
Console.WriteLine("{0},{1}", item.CategoryName, item.Description);
Console.ReadKey();
}
}
}
using System.Linq;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
CAContext context = new CAContext();
Categories entity;
//添加
entity = new Categories() { CategoryID = 0, CategoryName = "fruit", Description = "-", Picture = null };
context.Categories.Add(entity);
context.SaveChanges();
//修改
entity = context.Categories.Where(c => c.CategoryName == "fruit").First();
if (entity != null)
{
entity.Description = "fruit description.";
context.SaveChanges();
}
//删除
entity = context.Categories.Where(c => c.CategoryName == "fruit").First();
if (entity != null)
{
context.Categories.Remove(entity);
context.SaveChanges();
}
//查询
var categories = context.Categories;
foreach (var item in categories)
Console.WriteLine("{0},{1}", item.CategoryName, item.Description);
Console.ReadKey();
}
}
}
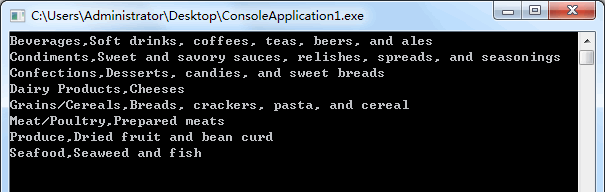
补充说明
[1].实体类主键必须有[Key]约束,否则程序运行会出错:

System.Data.Entity.Edm.EdmEntityType: : EntityType 'Categories' has no key defined. Define the key for this EntityType.
[2].如果实体类名和表名不一致,必须指定表名:
复制内容到剪贴板
程序代码

[Table("Categories")]
public class Categories2
{
[Key]
public int CategoryID { get; set; }
public string CategoryName { get; set; }
public string Description { get; set; }
public byte[] Picture { get; set; }
}
public class Categories2
{
[Key]
public int CategoryID { get; set; }
public string CategoryName { get; set; }
public string Description { get; set; }
public byte[] Picture { get; set; }
}
[3].实体类属性只能少于或等于表字段,否则添加记录会出错;






评论: 0 | 引用: 0 | 查看次数: 4050
发表评论
请登录后再发表评论!