不错呦!smile@林凯西,确保“准备文件”中的几个文件都有安装,S...您好,看了您这篇帖子觉得很有帮助。但是有个问题想请...我的修改过了怎么还被恶意注册呢 @jjjjiiii 用PJ快9年了,主要是A...PJ3啊,貌似很少有人用PJ了,现在不是WP就是z...@332347365,我当时接入时错误码没有-10...楼主,ChkValue值应为-103是什么意思呢?...大哥 你最近能看到我发的信息,请跟我联系,我有个制...
C#二维数组排列组合算法(每列取一个元素组合)
编辑:dnawo 日期:2016-08-19
复制内容到剪贴板
程序代码

/// <summary>
/// C#二维数组排列组合算法(每列取一个元素组合)
/// </summary>
/// <param name="args"></param>
/// <returns></returns>
static List<string> ArrayGroup(List<List<string>> args)
{
List<string> result = new List<string>();
int counter = 0;
for (int x = 0; x < args.Count; x++)
{
for (int y = 0; y < args[x].Count; y++)
{
//1.第1行直接添加
if (x == 0)
{
result.Add(args[x][y]);
}
//2.第N行(N>1)处理
else
{
//2.1 统计N-1行元素总数
if (y == 0)
{
counter = result.Count;
}
//2.2 y>0时,复制并添加元素
if (y > 0)
{
for (int z = 0; z < counter; z++)
{
result.Add(string.Format("{0}{1}", result[z], args[x][y]));
}
}
//2.3 y=0放最后来处理,避免声明临时变量存储result
if (y == args[x].Count - 1)
{
for (int z = 0; z < counter; z++)
{
result[z] = string.Format("{0}{1}", result[z], args[x][0]);
}
}
}
}
}
return result;
}
/// C#二维数组排列组合算法(每列取一个元素组合)
/// </summary>
/// <param name="args"></param>
/// <returns></returns>
static List<string> ArrayGroup(List<List<string>> args)
{
List<string> result = new List<string>();
int counter = 0;
for (int x = 0; x < args.Count; x++)
{
for (int y = 0; y < args[x].Count; y++)
{
//1.第1行直接添加
if (x == 0)
{
result.Add(args[x][y]);
}
//2.第N行(N>1)处理
else
{
//2.1 统计N-1行元素总数
if (y == 0)
{
counter = result.Count;
}
//2.2 y>0时,复制并添加元素
if (y > 0)
{
for (int z = 0; z < counter; z++)
{
result.Add(string.Format("{0}{1}", result[z], args[x][y]));
}
}
//2.3 y=0放最后来处理,避免声明临时变量存储result
if (y == args[x].Count - 1)
{
for (int z = 0; z < counter; z++)
{
result[z] = string.Format("{0}{1}", result[z], args[x][0]);
}
}
}
}
}
return result;
}
运行示例:
复制内容到剪贴板
程序代码

static void Main(string[] args)
{
List<List<string>> list = new List<List<string>>()
{
new List<string>() { "A", "B" },
new List<string>() { "a", "b", "c" }
};
List<string> result = ArrayGroup(list);
//result.Sort();
Console.WriteLine(string.Join(",", result.ToArray()));
Console.ReadKey();
}
{
List<List<string>> list = new List<List<string>>()
{
new List<string>() { "A", "B" },
new List<string>() { "a", "b", "c" }
};
List<string> result = ArrayGroup(list);
//result.Sort();
Console.WriteLine(string.Join(",", result.ToArray()));
Console.ReadKey();
}
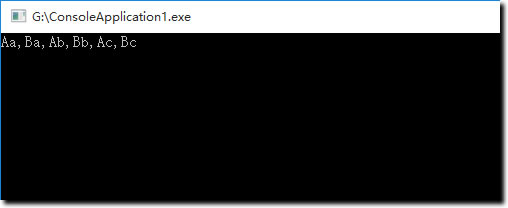
--------------------------------------------------------用Linq实现--------------------------------------------------------
复制内容到剪贴板
程序代码

static void Main(string[] args)
{
List<List<string>> list = new List<List<string>>()
{
new List<string>() { "A", "B" },
new List<string>() { "a", "b", "c" }
};
var result = list.Aggregate((m, n) => m.SelectMany(t1 => n.Select(t2 => t1 + t2).ToList()).ToList()).ToList();
Console.WriteLine(string.Join(",", result.ToArray()));
Console.ReadKey();
}
{
List<List<string>> list = new List<List<string>>()
{
new List<string>() { "A", "B" },
new List<string>() { "a", "b", "c" }
};
var result = list.Aggregate((m, n) => m.SelectMany(t1 => n.Select(t2 => t1 + t2).ToList()).ToList()).ToList();
Console.WriteLine(string.Join(",", result.ToArray()));
Console.ReadKey();
}
评论: 0 | 引用: 0 | 查看次数: 5083
发表评论
请登录后再发表评论!